Free Random Image API Services
- 10 May, 2023
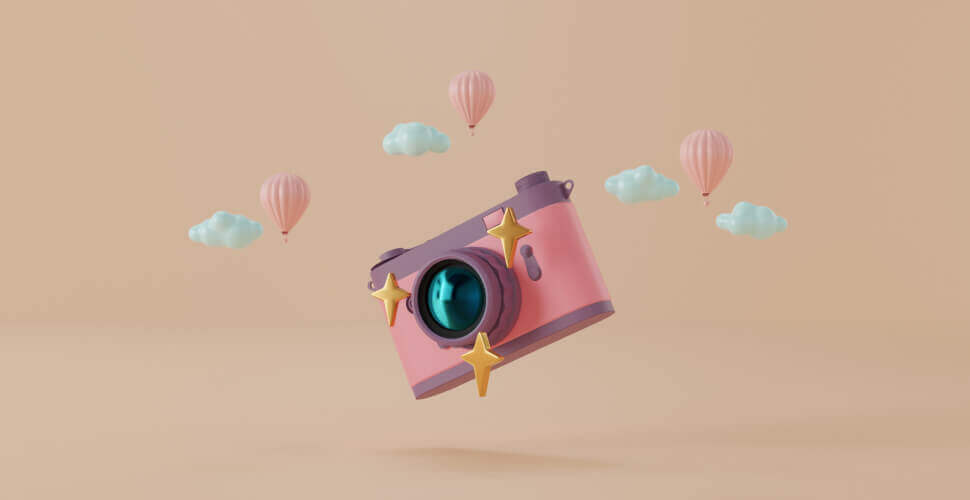
As a web developer, having access to quality image resources can significantly enhance the visual appeal of your projects. Random image APIs provide an excellent solution for dynamically adding images to websites, prototypes, or testing environments. In this post, I’ve compiled a list of free random image API services that you can integrate into your next project.
Why Use Random Image APIs?
Random image APIs offer several advantages:
- Save time searching for appropriate images
- Create more dynamic and engaging interfaces
- Easily implement placeholder images during development
- Test responsive layouts with various image dimensions
- Add visual interest to demos and prototypes
Top Random Image API Services
1. Unsplash Source API
For the most professional and high-quality photos, refer to the official Unsplash API documentation:
2. Picsum Photos (Lorem Picsum)
An excellent service providing high-quality stock photos:
https://picsum.photos/200/300
You can add parameters for grayscale or blur effects:
https://picsum.photos/200/300?grayscale
https://picsum.photos/200/300?blur=2
3. Ji Changxin API
Multiple image APIs for different use cases:
https://api.isoyu.com/bing_images.php // Daily Bing images
https://api.isoyu.com/mm_images.php // Female model images
4. Sakura API
Random anime-style wallpapers with JSON support:
https://www.dmoe.cc/random.php
https://www.dmoe.cc/random.php?return=json // JSON format
Implementation Examples
Basic HTML Image Tag
<img src="https://source.unsplash.com/random/800x600" alt="Random image">
Dynamic Background in CSS
.hero-section {
background-image: url('https://picsum.photos/1600/900');
background-size: cover;
height: 80vh;
}
Fetching Images with JavaScript
const updateRandomImage = () => {
const imgElement = document.getElementById('randomImg');
// Use Picsum Photos as a reliable option
imgElement.src = `https://picsum.photos/400/300?t=${new Date().getTime()}`;
};
document.getElementById('refreshBtn').addEventListener('click', updateRandomImage);
React Component Example
import { useState, useEffect } from 'react';
const RandomImage = ({ width = 400, height = 300 }) => {
const [imageSrc, setImageSrc] = useState('');
const refreshImage = () => {
// Add timestamp to prevent caching
setImageSrc(`https://picsum.photos/${width}/${height}?t=${Date.now()}`);
};
useEffect(() => {
refreshImage();
}, [width, height]);
return (
<div>
<img
src={imageSrc}
alt="Random visual"
width={width}
height={height}
style={{ objectFit: 'cover' }}
/>
<button onClick={refreshImage}>New Image</button>
</div>
);
};
export default RandomImage;
Considerations When Using These APIs
- Rate Limiting: Most free APIs have usage limits. Check documentation before implementing.
- Image Content: Some APIs may return unpredictable content. Test thoroughly before production.
- Performance: External APIs may affect page load times. Consider lazy loading.
- Reliability: Free services may experience downtime. Have fallback options.
- Content Licensing: Verify the license terms for any images used in production.
Conclusion
Random image APIs provide developers with convenient access to visual content for various projects. Whether you need placeholder images during development or dynamic content for production, these services offer flexible solutions to enhance your web applications.
For more serious projects, consider upgrading to premium API services that offer better reliability, content filtering, and higher rate limits.
What’s your favorite random image API? Have you implemented any of these in your projects? Share your experiences in the comments below!
Note: API availability and endpoints may change over time. Always check the official documentation for the most current information.